JavaScriptでモーダルウィンドウを表示させる方法【サンプル付きで解説】
PR ※当サイトではアフィリエイト広告を利用しています
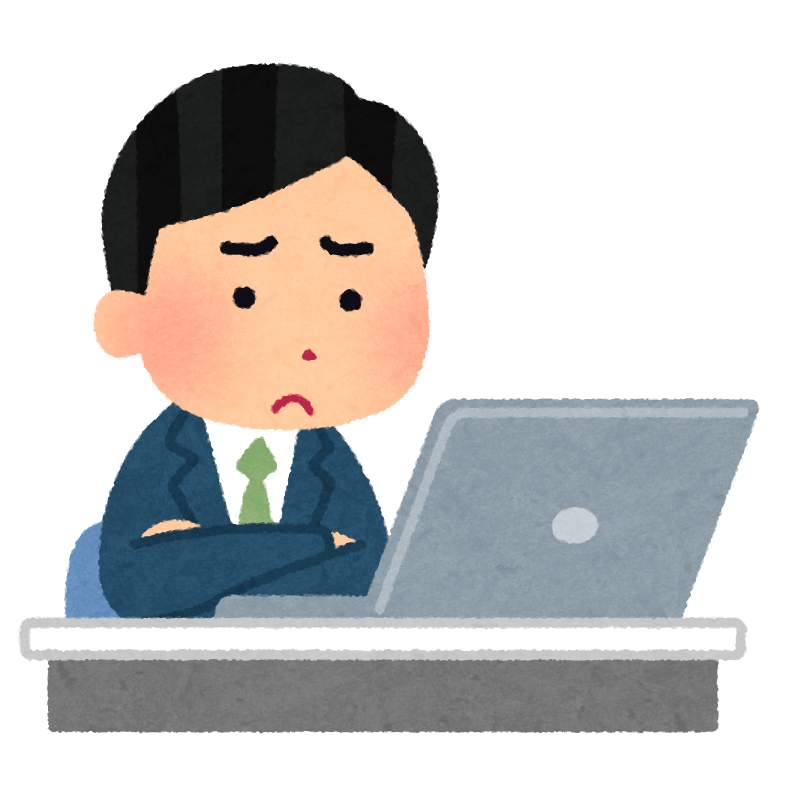
モーダルウィンドウを表示させたい人
「JavaScriptでモーダルウィンドウを表示させる方法を知りたい・・。何か良い方法があれば知りたいなぁ」
今回はJavaScriptでモーダルウィンドウを表示させる方法を紹介します。
おそらくjQueryで実装する人は多いかと思いますが、念のためにJavaScriptで作れるようにしておくと何かと便利だと思います。
JavaScriptでモーダルウィンドウを表示させる方法【サンプル付きで解説】
まずはこちらを見てください。
See the Pen
Untitled by かん @転職と副業とWeb制作 (@minimal_kan)
on CodePen.
HTMLで一行「buttonタグ」を記述して、あとはCSS・JavaScriptで出力しています。
それぞれコードの紹介をしていきます。
HTML
<body>
<button id="modal-button">タップして開く</button>
</body>
CSS
body {
background-size: cover;
background-color: #efefef;
}
.modal {
width: 100%;
height: 100%;
position: absolute;
top: 0;
left: 0;
background-color: rgba(0, 0, 0, 0.2);
display: flex;
justify-content: center;
align-items: center;
}
.modal .inner {
width: 100%;
height: 100%;
background-color: rgba(255, 255, 255, 0.9);
margin: 10px;
display: block;
width: 960px;
height: 540px;
border-radius: 5px;
-webkit-backdrop-filter: blur(16px);
box-shadow: 5px 5px 5px rgba(0, 0, 0, 0.3);
box-sizing: border-box;
padding: 20px;
text-align: center;
background-color: rgba(255, 255, 255, 0.9);
max-width: 600px;
max-height: 400px;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
color: #333;
font-size: 1.5rem;
animation: fadeInAnimation 200ms ease-out;
}
button{
background: #666;
color; #fff;
text-decoration: none;
color: #fff;
text-align: center;
border: none;
border-radius: 4px;
display: inline-block;
height: 72px;
line-height: 72px;
padding: 0 32px;
text-transform: uppercase;
font-size: 2rem;
cursor: pointer;
transition: background-color 0.2s;
}
button:hover{
background: #666;
opacity: 0.8;
}
@keyframes fadeInAnimation {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
CSSはJavaScriptで生成しているクラスをあらかじめ追記しておき、スタイルを整えています。
JavaScript
//クリックした時の処理
document.querySelector('#modal-button').addEventListener('click', openModalWindow);
//モーダルウィンドウを表示する
function openModalWindow(){
//モーダルを生成する→新しいタグを生成
const modalElement = document.createElement('div');
// 作ったdivの中にcss→modalを付与する
modalElement.classList.add('modal');
//モーダルウィンドウの中身を作る
//新しいdivタグを生成する
const innerElement = document.createElement('div');
//作ったdivの中にcss→innerを付与する
innerElement.classList.add('inner');
innerElement.innerHTML =
`<p>モーダルの中身です</p>
<p>テキストや画像も入るよ</p>`
;
//モーダルの中身に要素を配置する
modalElement.appendChild(innerElement);
//body要素にモーダルを配置する
document.body.appendChild(modalElement);
//中身をクリックしたらモーダルウインドウを削除する
innerElement.addEventListener('click',() => {
closeModalWindow(modalElement);
});
}
//モーダルウインドウを閉じる
function closeModalWindow(modalElement){
document.body.removeChild(modalElement);
}
クリックした時に「openModalWindow」が発火するようにしており、上から順番にそれぞれの動作を記述しています。
コード内にそれぞれの役割も記述しているので参考にしてみてください。
完成後は、下記のようにボタンを押すことで画面全体が背景色に覆われて中央にモーダルが表示されるようになりました。
シンプルなモーダルウィンドウ
他のパターンも紹介します。
こんな感じですね。
HTML
<button id="openModal">Open Modal</button>
<div id="myModal" class="modal">
<div class="modal-content">
<span id="closeModal">×</span>
<p>Some text in the Modal..</p>
</div>
</div>
CSS
.modal {
display: none;
position: fixed;
z-index: 1;
padding-top: 100px;
width: 100%;
height: 100%;
background-color: rgba(0,0,0,0.4);
}
.modal-content {
margin: auto;
padding: 20px;
border: 1px solid #888;
width: 80%;
background-color: #fff;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
JavaScript
var modal = document.getElementById("myModal");
var btn = document.getElementById("openModal");
var span = document.getElementById("closeModal");
btn.onclick = function() {
modal.style.display = "block";
}
span.onclick = function() {
modal.style.display = "none";
}
window.onclick = function(event) {
if (event.target == modal) {
modal.style.display = "none";
}
}
落ち着いたデザインのモーダルウィンドウ
ソースコードは上記からコピーしてください。
さまざまなカラーボタンのモーダルウィンドウ
ボタンの色は簡単に変更することができます。
モーダルウィンドウとは?特徴やコードを解説します
モーダルウィンドウとは、主にウェブサイトやアプリケーションで使用される特定のユーザインターフェース(UI)デザインパターンの一つです。
モーダルウィンドウは通常、親ウィンドウの上にポップアップ表示され、適切に使用すると非常に効果的なUI要素ですが、ユーザーのタスクの流れを中断するため、頻繁に使うとユーザー体験が悪くなります。そのため、必要なときだけ使用し、ユーザーが容易に閉じられるようにすることが大切です。
ほどよく使っていきましょう(`・ω・)ゞ
以上になります。
参考になったらぜひリツイートしてくれると嬉しいです。
リツイートする
合わせて読みたい記事